Get Started
Deploy Next.js with LaunchFlow
Deploy a Next.js application to AWS Fargate with LaunchFlow.
View the source code for this guide in our examples repo.
0. Set up your Next.js Project
If you already have a Next.js Project you can skip to step #1.
Create a new Next.js Application
1npx create-next-app@latest launchflow-nextjs
2cd launchflow-nextjs
Select all the default options.
Update src/app/page.tsx
to return a simple message:
1import { unstable_noStore as noStore } from "next/cache";
2
3export default function Home() {
4 // We use noStore here to allow us dynamic access of runtime environment variables (e.g. process.env.LAUNCHFLOW_ENVIRONMENT).
5 // If you don't need this feature you should remove this.
6 // See: https://nextjs.org/docs/app/building-your-application/configuring/environment-variables#runtime-environment-variables
7 noStore();
8 return <div>Hello from {process.env.LAUNCHFLOW_ENVIRONMENT}</div>;
9}
Update next.config.mjs
to output a standalone build:
1/** @type {import('next').NextConfig} */
2const nextConfig = {
3 output: "standalone",
4};
5
6export default nextConfig;
Create a Dockerfile
in the root of your project:
1FROM public.ecr.aws/docker/library/node:18-alpine AS base
2
3FROM base AS deps
4RUN apk add --no-cache libc6-compat
5WORKDIR /app
6
7COPY package.json yarn.lock* package-lock.json* pnpm-lock.yaml* ./
8RUN \
9 if [ -f yarn.lock ]; then yarn --frozen-lockfile; \
10 elif [ -f package-lock.json ]; then npm ci; \
11 elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm i --frozen-lockfile; \
12 else echo "Lockfile not found." && exit 1; \
13 fi
14
15FROM base AS builder
16WORKDIR /app
17COPY /app/node_modules ./node_modules
18COPY . .
19
20RUN \
21 if [ -f yarn.lock ]; then yarn run build; \
22 elif [ -f package-lock.json ]; then npm run build; \
23 elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm run build; \
24 else echo "Lockfile not found." && exit 1; \
25 fi
26
27FROM base AS runner
28WORKDIR /app
29
30ENV NODE_ENV=production
31
32RUN apk add --no-cache libcap
33RUN setcap 'cap_net_bind_service=+ep' /usr/local/bin/node
34RUN addgroup --system --gid 1001 nodejs
35RUN adduser --system --uid 1001 nextjs
36
37# NOTE: You may need to add this back if you have a public directory
38# COPY --from=builder /app/public ./public
39
40RUN mkdir .next
41RUN chown nextjs:nodejs .next
42
43COPY /app/.next/standalone ./
44COPY /app/.next/static ./.next/static
45
46USER nextjs
47
48EXPOSE 80
49ENV PORT=80
50
51ENV HOSTNAME="0.0.0.0"
52CMD ["node", "server.js"]
1. Initialize Launch Flow
If you're coming from a platform like Vercel you will need update two things:
- Add a Dockerfile to your project. If you don't have a Dockerfile you can get started with Next.js's recommended Dockerfile.
- Make sure your Next.js project is set to output a standalone build. You can do this by adding
output: "standalone"
to yournext.config.mjs
:
Install the LaunchFlow Python SDK and CLI using pip
.
1pip install launchflow[aws]
Initialize LaunchFlow in your project
1lf init --backend=local
- Name your project
- Select
Yes
for creating an exampleinfra.py
- Select
AWS
for your cloud provider - Select
ECS Fargate
for your service
Once finished you will get an infra.py
that looks like:
1import launchflow as lf
2
3# ECSFargateService Docs: https://docs.launchflow.com/reference/aws-services/ecs-fargate
4api = lf.aws.ECSFargateService(
5 "my-ecs-api",
6 dockerfile="Dockerfile", # Path to your Dockerfile
7)
ECSFargateService will build your Dockerfile and deploy to ECS Fargate. You can provide additional fields to ECSFargateService
to configure things like machine type, num instances, or even a custom domain.
2. Deploy your Service
Make sure you have local AWS credentials set up before deploying.
1lf deploy
- Name your environment (
dev
is a good first name) - Select your cloud provider
AWS
- Confirm the resources to be created
- Select the service to deploy
Once complete you will see a link to your deployed service on ECS Fargate.
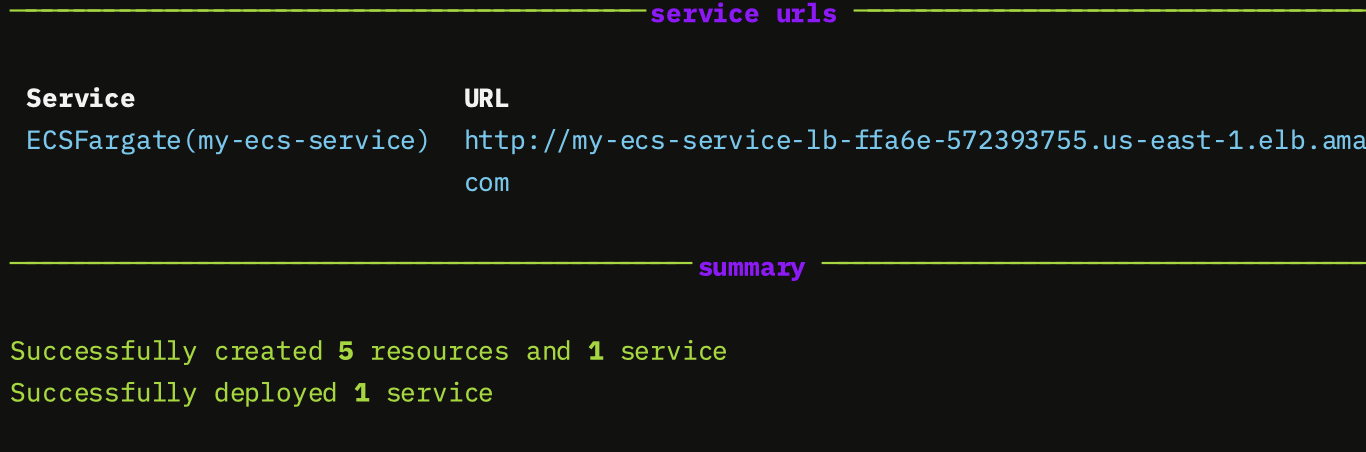
3. Cleanup your Resources
Optionally you can delete all your resources, service, and environments with:
1lf destroy
2lf environments delete
4. Visualize, Share, and Automate
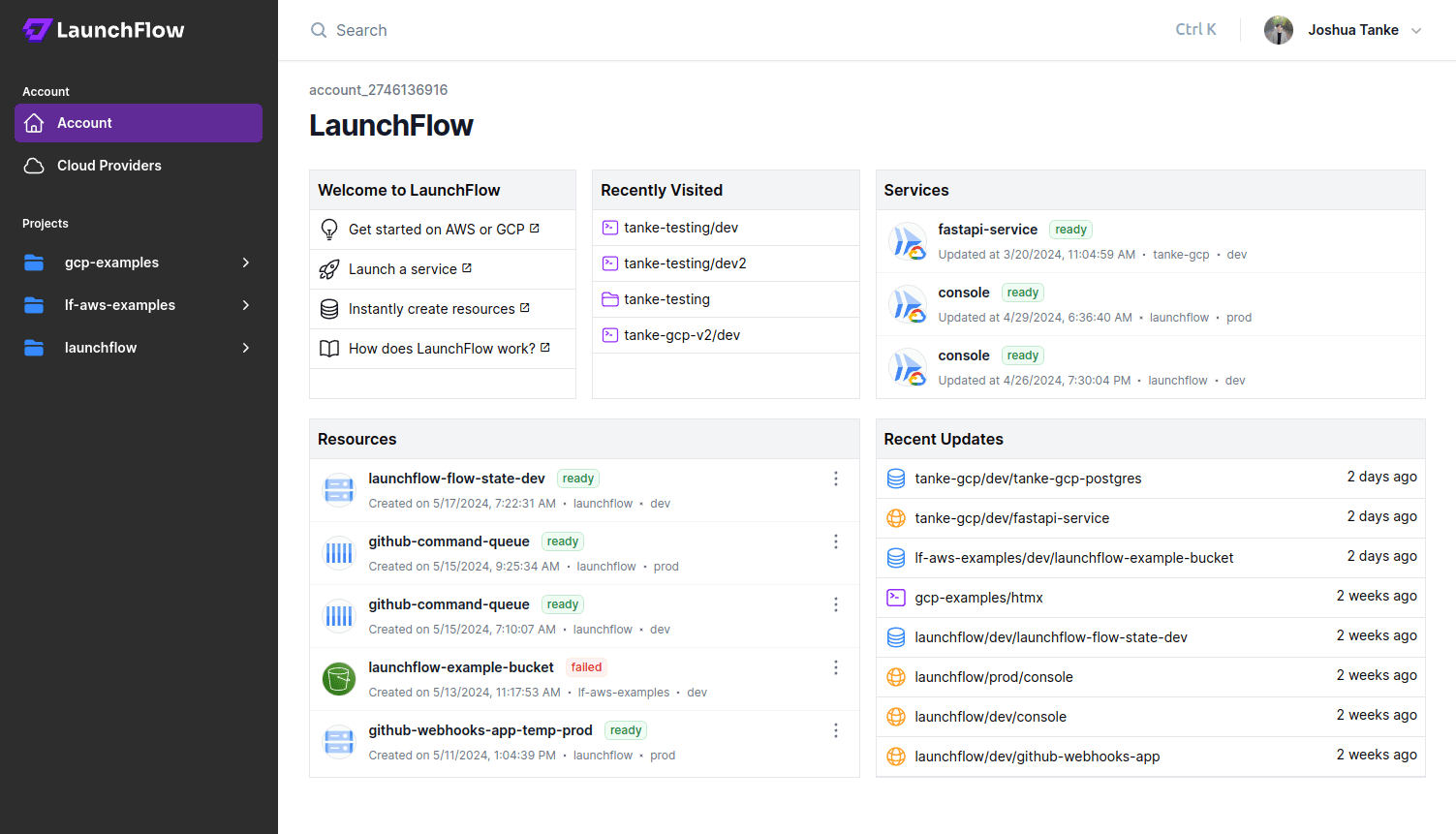
LaunchFlow Cloud usage is optional and free for individuals.
Using the local backend like we did above works fine for starting a project, but doesn't offer a way to share state between multiple users. LaunchFlow Cloud is a web-based service for managing, sharing, and automating your infrastructure. It's free small teams and provides a simple, secure way to collaborate with your team and automate your release pipelines.
Sign up for LaunchFlow Cloud and connect your local environment by running:
1lf init --backend=lf
This will create a project in your LaunchFlow Cloud account and migrate your local state to the LaunchFlow Cloud backend.
What's next?
- Add resources to your application
- Promote your application to a production enviroment
- Learn more about Environments, Services, and Resources
- Join theLaunchFlow Slack community
- View your application in theLaunchFlow console