Get Started
Deploy Spring Boot with LaunchFlow
Deploy a Spring Boot application to AWS Fargate with LaunchFlow.
View the source code for this guide in our examples repo.
0. Set up your Spring Boot Project
If you already have a Spring Boot Project you can skip to step #1.
Create a new Spring Boot Application
1spring init --dependencies=web launchflow-springboot
2cd launchflow-springboot
Update src/main/java/com/example/demo/DemoApplication.java
to include a simple REST controller:
1package com.example.demo;
2
3import org.springframework.boot.SpringApplication;
4import org.springframework.boot.autoconfigure.SpringBootApplication;
5import org.springframework.web.bind.annotation.GetMapping;
6import org.springframework.web.bind.annotation.RestController;
7
8@SpringBootApplication
9@RestController
10public class DemoApplication {
11
12 public static void main(String[] args) {
13 SpringApplication.run(DemoApplication.class, args);
14 }
15
16 @GetMapping("/")
17 public String hello() {
18 return "Hello from " + System.getenv("LAUNCHFLOW_ENVIRONMENT");
19 }
20}
Create a Dockerfile
in the root of your project:
1FROM public.ecr.aws/docker/library/openjdk:17-jdk-slim as build
2WORKDIR /workspace/app
3
4COPY mvnw .
5COPY .mvn .mvn
6COPY pom.xml .
7COPY src src
8
9RUN ./mvnw install -DskipTests
10RUN mkdir -p target/dependency && (cd target/dependency; jar -xf ../*.jar)
11
12FROM public.ecr.aws/docker/library/openjdk:17-jdk-slim
13WORKDIR /app
14VOLUME /tmp
15ARG DEPENDENCY=/workspace/app/target/dependency
16COPY ${DEPENDENCY}/BOOT-INF/lib /app/lib
17COPY ${DEPENDENCY}/META-INF /app/META-INF
18COPY ${DEPENDENCY}/BOOT-INF/classes /app
19
20ENV PORT=80
21EXPOSE $PORT
22ENTRYPOINT ["java","-cp","app:app/lib/*","com.example.demo.DemoApplication"]
1. Initialize Launch Flow
If you're deploying an existing app, ensure you have a Dockerfile in your project that builds and runs your Spring Boot application.
Install the LaunchFlow Python SDK and CLI using pip
.
1pip install launchflow[aws]
Initialize LaunchFlow in your project
1lf init --backend=local
- Name your project
- Select
Yes
for creating an exampleinfra.py
- Select
AWS
for your cloud provider - Select
ECS Fargate
for your service
Once finished you will get an infra.py
that looks like:
1import launchflow as lf
2
3# ECSFargateService Docs: https://docs.launchflow.com/reference/aws-services/ecs-fargate
4api = lf.aws.ECSFargateService(
5 "my-ecs-api",
6 dockerfile="Dockerfile", # Path to your Dockerfile
7)
ECSFargateService will build your Dockerfile and deploy to ECS Fargate. You can provide additional fields to ECSFargateService
to configure things like machine type, num instances, or even a custom domain.
2. Deploy your Service
Make sure you have local AWS credentials set up before deploying.
1lf deploy
- Name your environment (
dev
is a good first name) - Select your cloud provider
AWS
- Confirm the resources to be created
- Select the service to deploy
Once complete you will see a link to your deployed service on ECS Fargate.
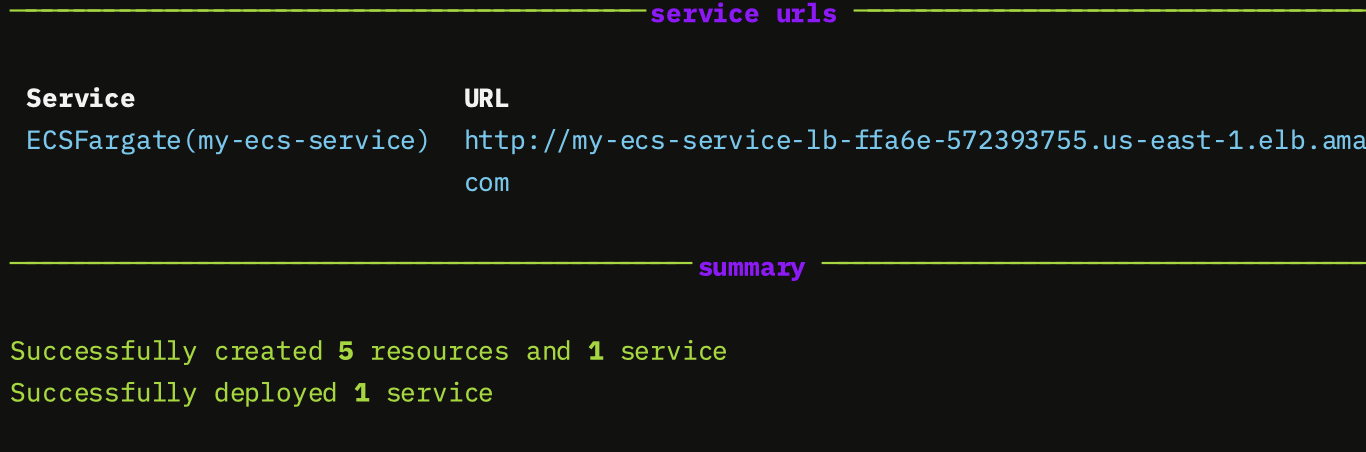
3. Cleanup your Resources
Optionally you can delete all your resources, service, and environments with:
1lf destroy
2lf environments delete
4. Visualize, Share, and Automate
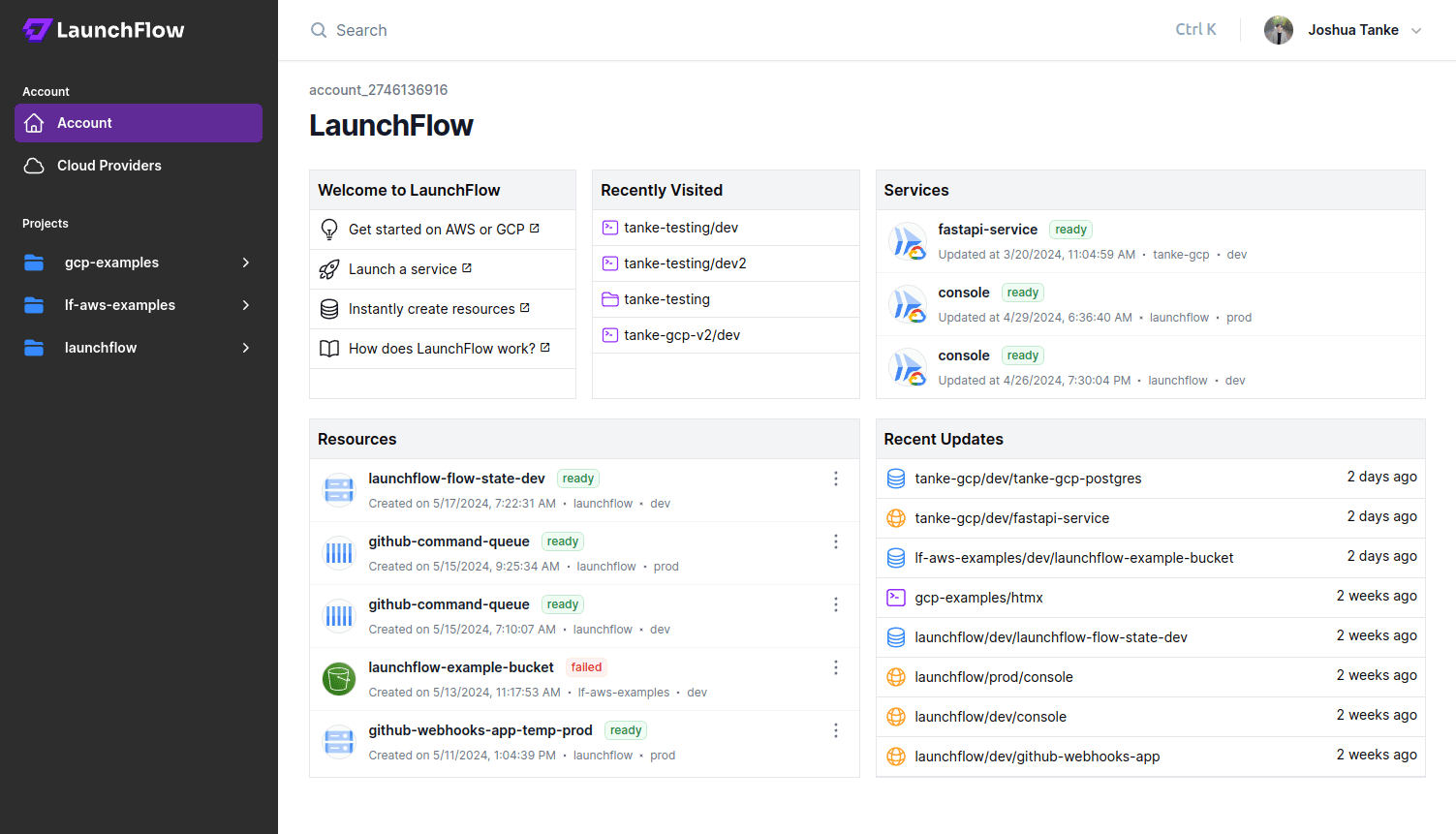
LaunchFlow Cloud usage is optional and free for individuals.
Using the local backend like we did above works fine for starting a project, but doesn't offer a way to share state between multiple users. LaunchFlow Cloud is a web-based service for managing, sharing, and automating your infrastructure. It's free small teams and provides a simple, secure way to collaborate with your team and automate your release pipelines.
Sign up for LaunchFlow Cloud and connect your local environment by running:
1lf init --backend=lf
This will create a project in your LaunchFlow Cloud account and migrate your local state to the LaunchFlow Cloud backend.
What's next?
- Add resources to your application
- Promote your application to a production enviroment
- Learn more about Environments, Services, and Resources
- Join theLaunchFlow Slack community
- View your application in theLaunchFlow console